C# Extension Method and Lambda Expressions
Recently I have been trying to learn more about the new language features in C# 3.0, and I have enjoyed what I have found thus far. Especially both the var keyword and extension methods, but I had yet to really implement anything using lambdas. That is until today...
One of the things I have done with extension methods was to implement some really sweet method argument validation stuff. I had read several related blog entries regarding this subject and had whipped up a variation [actually just a much smaller set of what they had already come up with as my needs were much less] of their ideas for use in a project I am working on [and likely in future projects]. And, as it turns out, this same bit of features afforded me the opportunity to check out lambda expressions as well, and to hopefully this time add something of value to build on their work instead of just reusing it.
Okay so the scene is set, with me at my desk at work, coding a unit test for what I hope will be a new feature on my current project [dynamically executed reports from xml definition files, like rdl but much much simpler]...
I was about to use the argument extension methods to validate an integer that I needed to be in a certain range and it occured to me how nice it would be if I could just pass in an expression that evaluated to a boolean result similar to what I would do if I was writing in javascript. Yeah, that would be extra nice! So, off I went back to GOG to do some research on passing an expression as an argument to a function in C#. My research led me straight to lambda expressions and exactly what I needed to make my new extension method work.
My goal was to be able to implement something like the following psuedo-code:
public void MyFunction(int myInt)
{
RequireThat(myInt).MeetsCriteria("myInt > 0");
}
Thus being able to use some very smooth and descriptive code to validate my integer argument before using it in the function, or atleast something as close to that effect as I could get.
Well lambda expressions were exactly the ticket, specifically the Func<T, TResult> delegate type. Which basically allows me to pass a method that accepts one parameter of type T and returns a result of type TResult as a parameter to another method, without defining a custom delegate type of my own. A kinda anonymous delegate type construct if you will. Lambda expressions use this type as an arg for the Expression<T>(Func<T, TResult) type constructor. I have done some of this preliminary reading on Expression Trees and such and it is heady stuff, but interesting none the less. I look forward to someday being able to apply it to a real world problem.
But today I was able to apply lambdas to my real world problem like so:
I needed an extension method for my Generic argument wrapper that would allow me to pass in a simple expression predicate with which to validate the argument. And here is what I came up with:
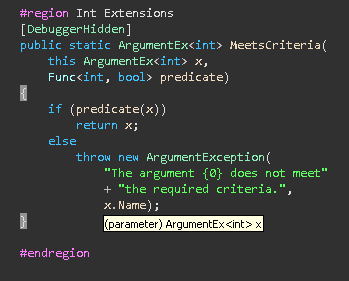
Now this code allows me to pass in a lambda expression that I wish to use to validate an integer argument for my method [or any integer for that matter]. This method was added to my existing argument validation extension methods setup as described in a previous post, already linked to a couple of times above, so it follows similar usage syntax, as such:
myIntArg.RequireThat(<span style="color:#800000;">"myIntArg"</span>).MeetsCriteria(...);
I also added a unit test for this new method into my existing test project for the rest of my validation extension methods, and so I will use that test method to show you a contextual usage of MeetsCriteria...
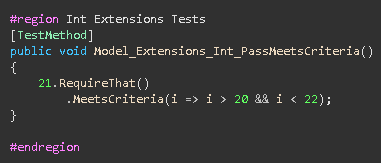
So, now I have actually used lambdas in a project at work, I am so very happy with myself. And my quest to conquer C# 3.0 features continues... J